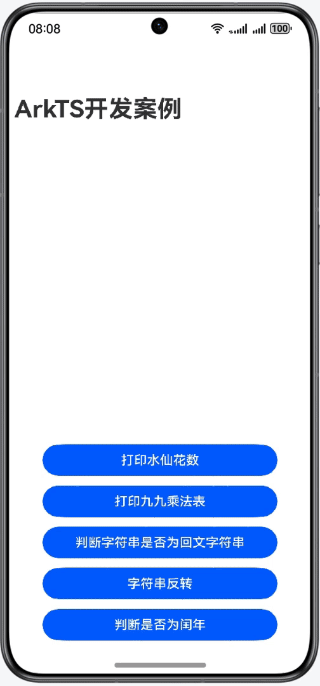
案例地址
代码结构
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 |
├──entry/src/main/ets/ │ ├──common │ │ ├──constants │ │ │ └──Constants.ets // 常量类 │ │ └──utils │ │ ├──CommonUtils.ets // 弹窗工具类 │ │ ├──Logger.ets // 日志工具类 │ │ └──Method.ets // 算法工具类 │ ├──entryability │ │ └──EntryAbility.ets │ ├──entrybackupability │ │ └──EntryBackupAbility.ets │ ├──pages │ │ └──Index.ets // 界面实现 │ └──view │ ├──DaffodilsNumberCustomDialog.ets // 水仙花数弹窗实现 │ ├──IsLeapYearCustomDialog.ets // 闰年判断数弹窗实现 │ ├──IsPalindromicStringCustomDialog.ets // 回文字符串判断数弹窗实现 │ ├──MultiplicationTableCustomDialog.ets // 九九乘法表弹窗实现 │ └──StringReversalCustomDialog.ets // 字符串反转弹窗实现 └───entry/src/main/resources // 应用资源目录 |
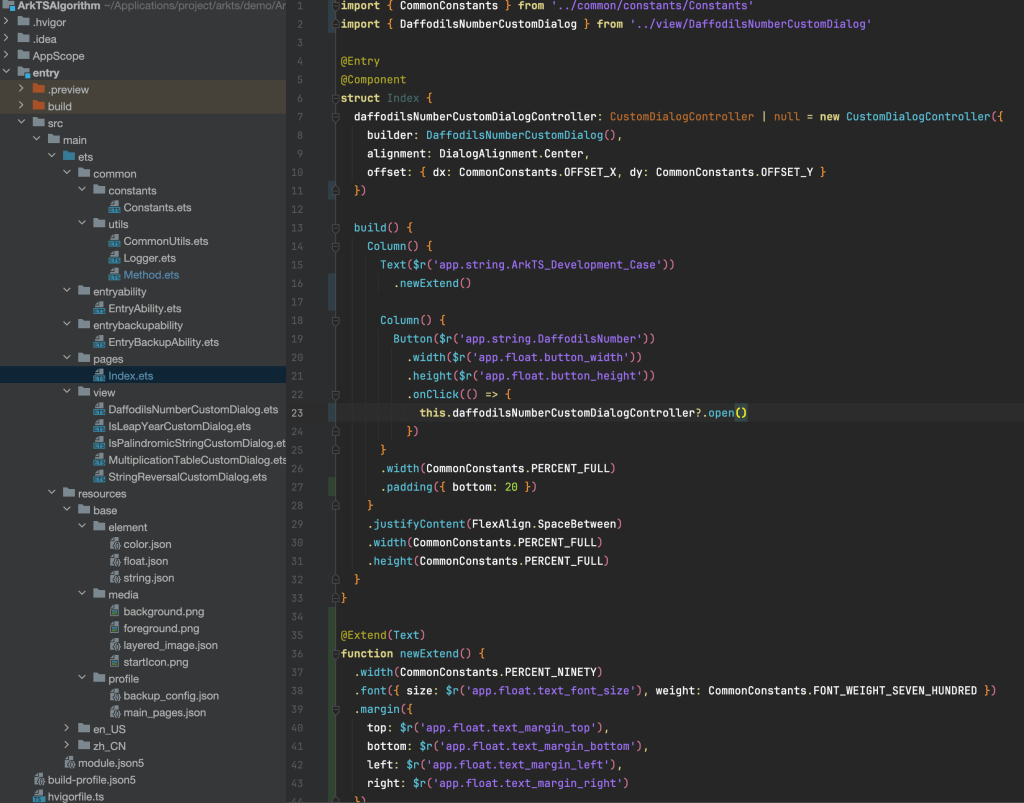
代码
Index.ets
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 |
import { CommonConstants } from '../common/constants/Constants' import { DaffodilsNumberCustomDialog } from '../view/DaffodilsNumberCustomDialog' @Entry @Component struct Index { daffodilsNumberCustomDialogController: CustomDialogController | null = new CustomDialogController({ builder: DaffodilsNumberCustomDialog(), alignment: DialogAlignment.Center, offset: { dx: CommonConstants.OFFSET_X, dy: CommonConstants.OFFSET_Y } }) build() { Column() { Text($r('app.string.ArkTS_Development_Case')) .newExtend() Column() { Button($r('app.string.DaffodilsNumber')) .width($r('app.float.button_width')) .height($r('app.float.button_height')) .onClick(() => { this.daffodilsNumberCustomDialogController?.open() }) } .width(CommonConstants.PERCENT_FULL) .padding({ bottom: 20 }) } .justifyContent(FlexAlign.SpaceBetween) .width(CommonConstants.PERCENT_FULL) .height(CommonConstants.PERCENT_FULL) } } @Extend(Text) function newExtend() { .width(CommonConstants.PERCENT_NINETY) .font({ size: $r('app.float.text_font_size'), weight: CommonConstants.FONT_WEIGHT_SEVEN_HUNDRED }) .margin({ top: $r('app.float.text_margin_top'), bottom: $r('app.float.text_margin_bottom'), left: $r('app.float.text_margin_left'), right: $r('app.float.text_margin_right') }) } |
Contants.ets
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 |
/** * Common constants for all features. */ export class CommonConstants { /** * String for input content. */ static readonly OFFSET_X: number = 0; /** * String for input content. */ static readonly OFFSET_Y: number = -25; /** * Number for zip level. */ static readonly PERCENT_NINETY: string = '90%'; /** * Number for zip level. */ static readonly PERCENT_FULL: string = '100%'; /** * Number for zip level. */ static readonly FONT_WEIGHT_SEVEN_HUNDRED: number = 700; /** * Number for zip level. */ static readonly LEAP_YEAR_INPUT: string = '请输入一个年份'; /** * Number for zip level. */ static readonly LEAP_YEAR_YES: string = '该年份是闰年'; /** * Number for zip level. */ static readonly LEAP_YEAR_NO: string = '该年份不是闰年'; /** * Number for zip level. */ static readonly STRING_INPUT: string = '请输入一个字符串'; /** * Number for zip level. */ static readonly PALINDROMIC_STRING_YES: string = '该字符串是回文字符串'; /** * Number for zip level. */ static readonly PALINDROMIC_STRING_NO: string = '该字符串不是回文字符串'; /** * Number for zip level. */ static readonly STRING_PRE: string = '反转结果为:'; /** * The time of toast */ static readonly TOAST_TIME: number = 2000; } |
Method.ets
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 |
export function daffodilsNumber(): number[] { let result: number[] = [] for (let i = 100; i < 1000; i++) { let unitsDigit: number = i % 10 let tenthsDigit: number = Math.floor(i / 10) - Math.floor(i / 100) * 10 let hundredthsDigit: number = Math.floor(i / 100) if (i === unitsDigit * unitsDigit * unitsDigit + tenthsDigit * tenthsDigit * tenthsDigit + hundredthsDigit * hundredthsDigit * hundredthsDigit) { result.push(i) } } return result } export function multiplicationTable(): string[][] { let result: string[][] = []; for (let i = 1; i <= 9; i++) { let index: string[] = []; for (let j = 1; j <= i; j++) { let temp: string = j + ' * ' + i + ' = ' + i * j; index.push(temp); } result.push(index); } return result; } export function isPalindromicString(content: string): boolean { let result: boolean = true; let i: number = 0; let j: number = content.length - 1; while (i <= j) { if (content.charAt(i) !== content.charAt(j)) { result = false; break; } i++; j--; } return result; } export function stringReversal(content: string): string { let result: string = ''; for (let index = content.length - 1; index >= 0; index--) { result += content.charAt(index); } return result; } export function isLeapYear(year: number): boolean { let result: boolean = false; if ((year % 4 === 0 && year % 100 !== 0) || (year % 400 === 0)) { result = true; } else { result = false; } return result; } |
DaffodilsNumberCustomDialog.ets
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 |
import { CommonConstants } from '../common/constants/Constants'; import { daffodilsNumber } from '../common/utils/Method'; @CustomDialog export struct DaffodilsNumberCustomDialog { IsPalindromicStringCustomDialogController?: CustomDialogController; build() { Column() { Column() { Text($r('app.string.ArkTS_Development_Case')) .height($r('app.float.button_height')) .font({ size: $r('sys.float.ohos_id_text_size_headline8') }) .fontColor($r('sys.color.ohos_id_color_text_primary')) .margin({ top: $r('app.float.dialog_text_margin_top') }) Text($r('app.string.DaffodilsNumberTitle')) .font({ size: $r('sys.float.ohos_id_text_size_body2') }) .fontColor($r('sys.color.ohos_id_color_text_secondary')) .margin({ left: $r('app.float.dialog_text_margin_left') }) } .alignItems(HorizontalAlign.Center) .width(CommonConstants.PERCENT_FULL) .height($r('app.float.dialog_text_height')) Text(daffodilsNumber().toString()) .font({ size: $r('sys.float.ohos_id_text_size_body1') }) .fontColor($r('sys.color.ohos_id_color_text_primary')) .margin({ top: $r('app.float.dialog_padding') }) } .alignItems(HorizontalAlign.Center) .padding({ left: $r('app.float.dialog_padding'), right: $r('app.float.dialog_padding'), bottom: $r('app.float.dialog_padding') }) } } |
float.json
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 |
{ "float": [ { "name": "text_margin_top", "value": "64vp" }, { "name": "text_margin_bottom", "value": "8vp" }, { "name": "text_margin_left", "value": "12vp" }, { "name": "text_margin_right", "value": "20vp" }, { "name": "text_font_size", "value": "30fp" }, { "name": "button_width", "value": "288vp" }, { "name": "button_height", "value": "40vp" }, { "name": "button_margin_top", "value": "12vp" }, { "name": "button_margin_bottom", "value": "16vp" }, { "name": "dialog_text_margin_left", "value": "10vp" }, { "name": "dialog_text_height", "value": "72vp" }, { "name": "dialog_text_margin_top", "value": "8vp" }, { "name": "dialog_padding", "value": "24vp" }, { "name": "dialog_button_width", "value": "296vp" }, { "name": "dialog_textInput_height", "value": "48vp" } ] } |